Globally Disable TLS Checks with Java for HttpsURLConnection
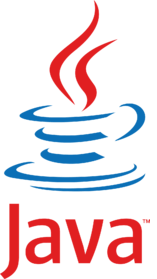
I am going to preface this article with a very strong note that this is not a good idea. It is horribly insecure, and will cause you problems if used without really contemplating the repercussions.
Today I've been integrating fusionauth-jwt into jwks-ical. Because I don't know how folks are going to have their certificates set up, I wanted to ensure that TLS validation was disabled when I first set up the project.
As fusionauth-jwt provides no hooks into it to customise the HttpsURLConnection
, I needed some way to globally configure this.
By adapting this answer from StackOverflow, we are able to globally set it, so when the library reaches through to create a new connection it will have certificate validation disabled:
import java.security.SecureRandom;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.X509TrustManager;
private void trustEveryone() {
try {
SSLContext context = SSLContext.getInstance("TLS");
HttpsURLConnection.setDefaultHostnameVerifier(
(hostname, session) -> true);
context.init(
null,
new X509TrustManager[] {
new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType)
throws CertificateException {}
public void checkServerTrusted(X509Certificate[] chain, String authType)
throws CertificateException {}
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
}
},
new SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(context.getSocketFactory());
} catch (Exception e) {
e.printStackTrace();
}
}