Sorting a Hash Recursively with Ruby
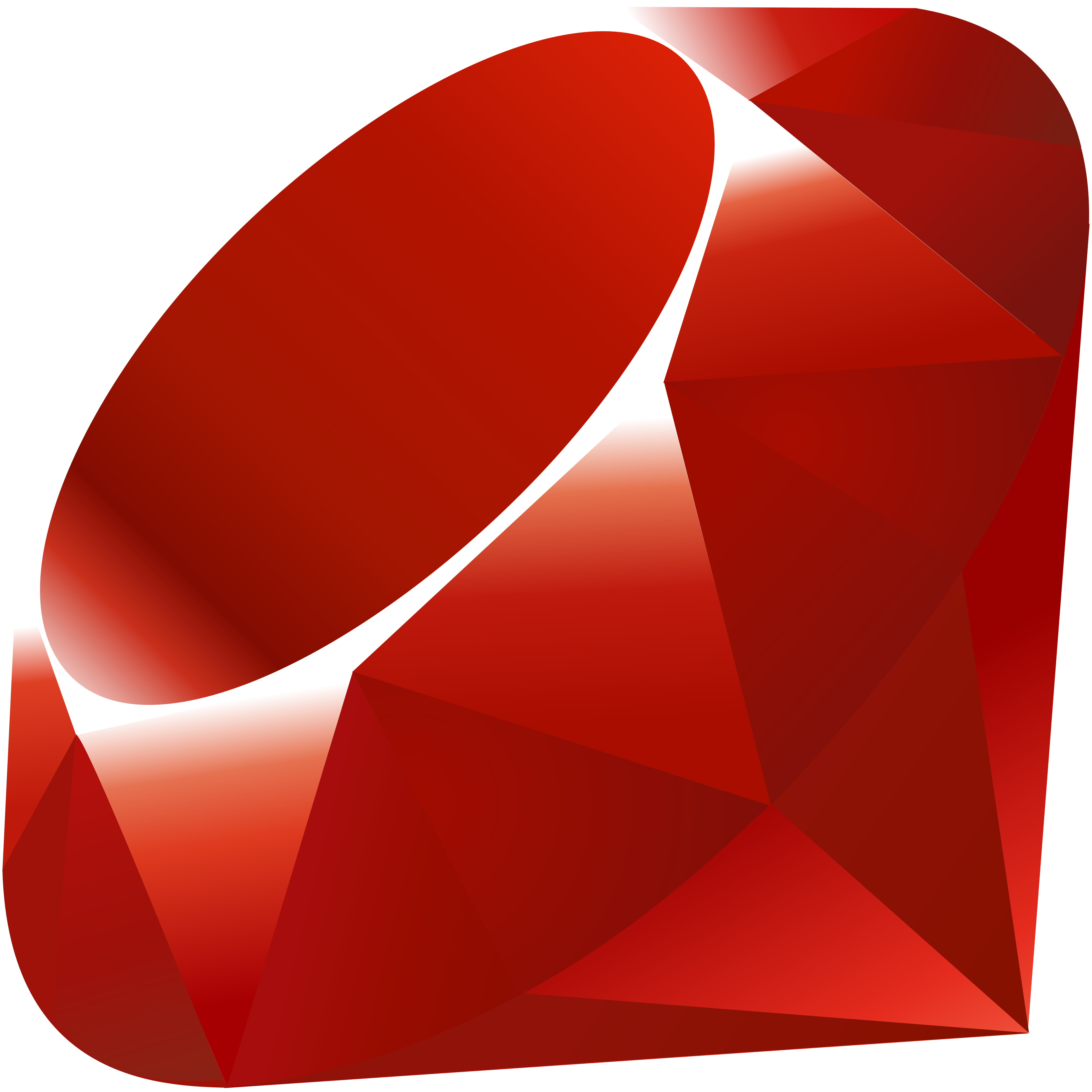
As part of Diffing Pretty-Printed JSON Files, I wanted to have a method of making JSON documents easier to diff by sorting their keys.
I wanted to use this solution by Brian Dunagan, but it didn't seem to quite work when, in my example, I had an Array
s which may have Hash
es but may also not.
My amended solution is:
def recursive_sort(v, &block)
if v.class == Hash
v.sorted_hash(&block)
elsif v.class == Array
v.collect {|a| recursive_sort(a, &block)}
else
v
end
end
class Hash
def sorted_hash(&block)
self.class[
self.each do |k,v|
self[k] = recursive_sort(v, &block)
end.sort(&block)]
end
end