Converting an Image to a Base64 data
URL with Node.JS
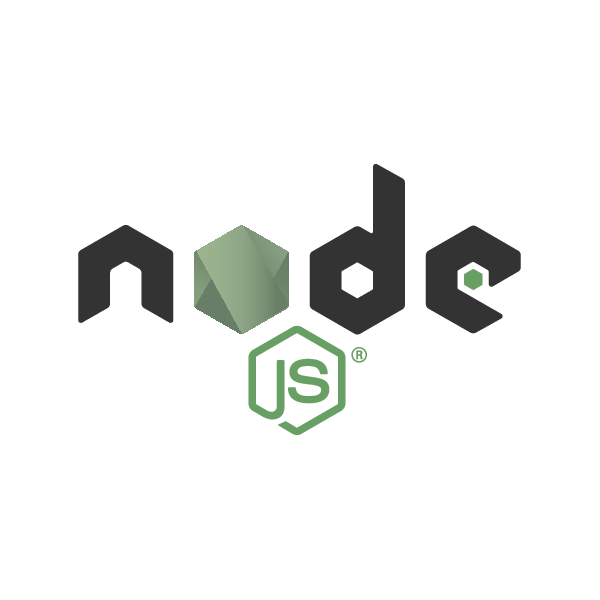
Data URLs are a pretty handy way of encoding small files, or binary data, into HTML.
Sometimes you'll want to use them for adding images inline into an HTML file, so will want a handy way of converting the image to the correctly formatted URL, of the form:
data:image/png;base64,....
A "good enough" script is to use the following, which fits into a oneliner:
node -e 'console.log(`data:image/png;base64,${fs.readFileSync(process.argv[1], "base64")}`)' /path/to/image
Or as a standalone script:
// node image.js /path/to/image
const fs = require('fs')
const contents = fs.readFileSync(process.argv[2], "base64")
console.log(`data:image/png;base64,${contents}`)
Note that we've hardcoded image/png
- Firefox (v91 Nightly, at the time of writing) allows either a JPEG or a PNG to be provided as such, which is great, as does Chromium (v96), but this isn't the best solution as it may not always work.
A better solution is that we use the image-type
library to correctly determine the media type for the provided file:
const fs = require('fs')
const imageType = require('image-type')
const contents = fs.readFileSync(process.argv[2])
const b64 = contents.toString('base64')
const type = imageType(contents)
if (type.mime === null) {
process.exit(1)
}
console.log(`data:${type.mime};base64,${b64}`)
Which can then be run as:
node image.js /path/to/image