Generate Plain Old Java Objects (POJOs) from OpenAPI Model Definitions with Gradle
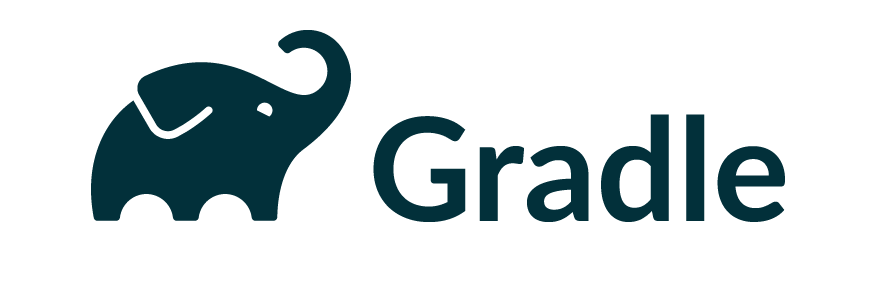
It's possible that you're using OpenAPI specifications to describe the format of your API.
If you're on a Java project, it may be that you're currently manually translating from OpenAPI data types to Plain Old Java Objects (POJOs), which is time consuming and has the risk of drifting between your definitions and implementations.
One of the benefits of using a very structured format like the format in OpenAPI specifications is that you can programmatically generate the contents of your Java models, or any other languages you may be using, for that matter.
Example OpenAPI specification
We'll base this on the OpenAPI specification demo from the Petstore, but this also works with OpenAPI specs that have $ref
s out to JSON schema documents, too!
Generating the POJOs
We can utilise the openapi-generator Gradle plugin to generate this for us, using the following Gradle configuration:
plugins {
id 'java'
id 'org.openapi.generator' version '5.4.0'
}
openApiGenerate {
generatorName = "java"
inputSpec = "$rootDir/specs/petstore.yaml"
outputDir = "$buildDir/generated/sources/openapi"
modelPackage = "io.swagger.petstore3.api.models"
generateModelTests = false // personal preference, as it's JUnit 4
globalProperties = [
// force only the models
apis: "false",
invokers: "false",
models: "",
]
configOptions = [
dateLibrary: "java8",
]
}
compileJava.dependsOn tasks.openApiGenerate
sourceSets.main.java.srcDirs += "$buildDir/generated/sources/openapi/src/main/java"
This is a bit stricter to only generate the classes for models, but we can also generate other API metadata such as an autogenerated client for interacting with the API!
This by default will generate a set of GSON models, but if you're i.e. using Spring Boot you'd want:
openApiGenerate {
configOptions = [
library: "resttemplate", // for example
]
}