Capturing HTTP Requests with okhttp's MockWebServer.takeRequest
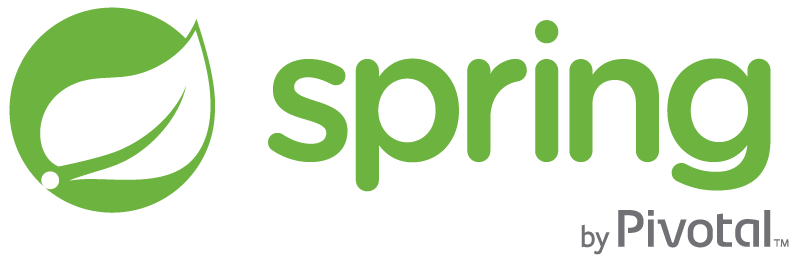
I came across okhttp's MockWebServer
as part of Integration Testing Your Spring WebClient
s with okhttp's MockWebServer
, and wanted to verify that HTTP requests made by my HTTP clients were well-formed.
To do this, there's the takeRequest
method, which on my first read, I expected to need to be called at the same time as the HTTP request was outgoing.
It turns out that the way to do this, is instead to call it after an HTTP request is sent, using it purely for verification means:
@Test
void test() throws InterruptedException {
MockWebServer server = new MockWebServer();
// required to be set, otherwise `takeRequest` will never return anything
server.enqueue(new MockResponse());
RestAssured.given().get(server.url("/products").toString());
RecordedRequest request = server.takeRequest(1, TimeUnit.SECONDS);
assertThat(request).isNotNull();
assertThat(request.getPath()).isEqualTo("/products");
}
Note the need for enqueueing a MockResponse
, as without it the tests will fail.