Reading all of stdin
on the command-line with Go
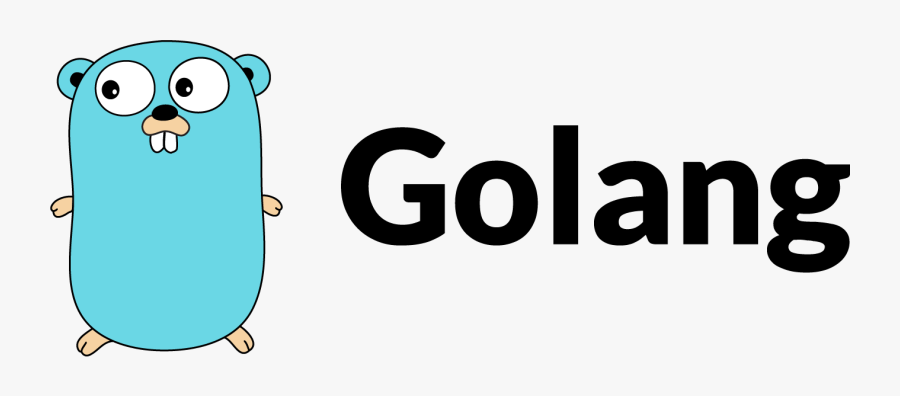
If you're writing command-line tools with Go, you're very likely to want to parse all of stdin
, from the first byte, up until the End of File character (EOF).
For instance, you may be receiving input from various means:
# piping from a file
go run main.go < file.txt
# piping with a heredoc
go run main.go <<< EOF
Multi-line
heredoc
EOF
# useless use of cat, but example of piping a command
cat file.txt | go run main.go
# writing the text to stdin
go run main.go
example
text
^D
To read this, in its entirety, we can use io.ReadAll
like so:
package main
import (
"fmt"
"io"
"os"
"strings"
)
func main() {
// this does all the work for us!
stdin, err := io.ReadAll(os.Stdin)
if err != nil {
panic(err)
}
str := string(stdin)
fmt.Println(strings.TrimSuffix(str, "\n"))
}
As noted above, this comment from 2018 noting that you stdin
doesn't close no longer seems to be the case, at least in the test I performed on go version go1.17.7 linux/amd64
.