Converting Spring Boot Property Variables to Environment Variables with Go
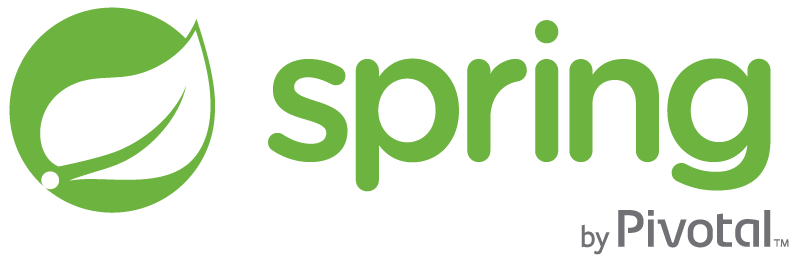
Since I migrated my personal Spring Boot applications to run on Kubernetes, I've been using Spring Boot's environment variables support to configure the application.
After doing this manually for a while, I've found that it's time to automate it, so I've reached for Go as a means to provide a handy command-line program:
package main
import (
"fmt"
"io"
"os"
"regexp"
"strings"
)
func convert(s string) string {
s = strings.ReplaceAll(s, ".", "_")
s = strings.ReplaceAll(s, "-", "")
listRe := regexp.MustCompile(`\[([0-9]+)\]`)
s = listRe.ReplaceAllString(s, "_${1}_")
s = strings.ReplaceAll(s, "__", "_")
return strings.ToUpper(s)
}
func main() {
data, err := io.ReadAll(os.Stdin)
if err != nil {
panic(err)
}
propertyAndValue := strings.Split(strings.TrimSuffix(string(data), "\n"), "=")
if len(propertyAndValue) == 1 {
fmt.Println(convert(propertyAndValue[0]))
} else {
fmt.Println(convert(propertyAndValue[0]) + "=" + propertyAndValue[1])
}
}
That can be run like so, handling the property's value, as well as list-based configuration:
$ go run main.go <<< spring.main.log-startup-info
SPRING_MAIN_LOGSTARTUPINFO
$ go run main.go <<< spring.main.log-startup-info=1234.foo.bar
SPRING_MAIN_LOGSTARTUPINFO=1234.foo.bar
$ go run main.go <<< my.service[0].other
MY_SERVICE_0_OTHER