Testing Go net/http
handlers
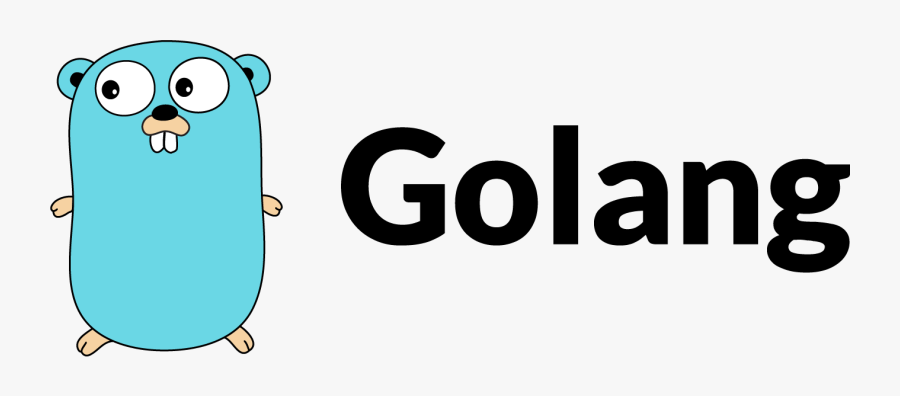
When writing Go web services, it's very likely that you'll be unit testing the HTTP handlers, as well as doing some wider integration tests on your application.
Something nice about Go is the httptest package in the standard library, providing a very handy means to test HTTP logic.
This means that if we had the following, very straightforward handler:
func Handler(w http.ResponseWriter, r *http.Request) {
w.Header().Add("tracing-id", r.Header.Get("tracing-id"))
w.WriteHeader(401)
}
We can then write the following unit test:
func Test_Handler_status(t *testing.T) {
rr := httptest.NewRecorder()
req := httptest.NewRequest("GET", "/apis", nil)
req.Header.Add("tracing-id", "123")
Handler(rr, req)
if rr.Result().StatusCode != 401 {
t.Errorf("Status code returned, %d, did not match expected code %d", rr.Result().StatusCode, 401)
}
if rr.Result().Header.Get("tracing-id") != "123" {
t.Errorf("Header value for `tracing-id`, %s, did not match expected value %s", rr.Result().Header.Get("tracing-id"), "123")
}
}