Getting all comments from a given user on a GitHub Discussion
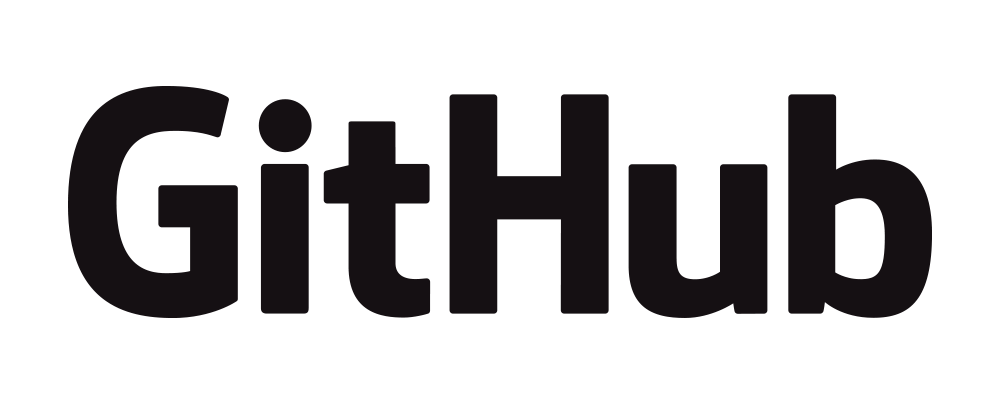
As noted previously, my team practices asynchronous, always-updating standups through GitHub Discussions.
As it's performance management season, I'm looking back at what I've done over the last few months, and wanted to get a view of all the standup updates I've made, having spent far too long trying to do it manually, and then pop them into a Markdown file that I could then reference.
To do this, I've crafted the following GraphQL API query:
query ($owner: String!, $repo: String!, $discussionNumber: Int!) {
repository(owner: $owner, name: $repo) {
discussion(number: $discussionNumber) {
comments(last: 100) {
edges {
node {
body
replies(last: 100) {
edges {
node {
databaseId
body
createdAt
author {
login
}
}
}
}
}
}
}
}
}
}
To make it simpler, I've wrapped this in the GitHub CLI, gh
, and used a small Ruby script to post-process:
require 'json'
def exit_usage
puts 'Usage: discussion-comments.rb $owner $repo $discussion_id'
exit 1
end
query = '
query ($owner: String!, $repo: String!, $discussionNumber: Int!) {
repository(owner: $owner, name: $repo) {
discussion(number: $discussionNumber) {
comments(last: 100) {
edges {
node {
body
replies(last: 100) {
edges {
node {
databaseId
body
createdAt
author {
login
}
}
}
}
}
}
}
}
}
}
'
owner = ARGV[0]
repo = ARGV[1]
id = ARGV[2]
user = ARGV[3]
exit_usage unless owner
exit_usage unless repo
exit_usage unless id
exit_usage unless user
out = `gh api graphql -f query='#{query}' -F owner=#{owner} -F repo=#{repo} -F discussionNumber=#{id}`
data = JSON.parse(out)
data['data']['repository']['discussion']['comments']['edges'].each do |edge|
edge['node']['replies']['edges'].each do |reply|
next unless reply['node']['author']['login'] == user
puts "## #{reply['node']['createdAt']}"
puts ''
puts reply['node']['body']
puts ''
puts ''
end
end
I've purposefully not invested too much time in error handling - maybe one for next quarter's performance review?