Performing group_concat
queries over distinct values, with a custom delimiter, with SQLite
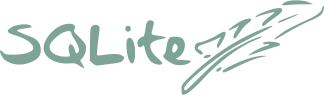
Let's say that we have the following query:
select
package_name,
version,
count(*),
group_concat(distinct owner) as owners
from
renovate
left join owners on renovate.platform = owners.platform
and renovate.organisation = owners.organisation
and renovate.repo = owners.repo
where
package_name like 'github.com/oapi-codegen/%'
group by
package_name, version
order by
version asc
This may output something like:
+------------------------------------+---------+----------+-----------------------------------------------------+
| PACKAGE NAME | VERSION | COUNT(*) | OWNERS |
+------------------------------------+---------+----------+-----------------------------------------------------+
| github.com/deepmap/oapi-codegen | v1.12.4 | 2 | team-one,team-two,another-team,what-about-us |
+------------------------------------+---------+----------+-----------------------------------------------------+
This isn't the best output, especially if you're presenting it to humans and there's a long comma-separated list of values.
To make this a little nicer, we'd want to use a delimiter, which is available as an argument to the group_concat
function.
For instance, let's add a newline delimiter to split our owner
s:
select
package_name,
version,
count(*),
-- this line is modified
group_concat(distinct owner, '
') as owners
from
renovate
left join owners on renovate.platform = owners.platform
and renovate.organisation = owners.organisation
and renovate.repo = owners.repo
where
package_name like 'github.com/oapi-codegen/%'
group by
package_name, version
order by
version asc
Frustratingly, this errors:
Parse error: DISTINCT aggregates must have exactly one argument
Via this StackOverflow question, we can instead use the replace
function to replace the default comma-separated delimiter with our custom one, like so:
select
package_name,
version,
count(*),
-- this line is modified
replace(group_concat(distinct owner), ',', '
') as owners
from
renovate
left join owners on renovate.platform = owners.platform
and renovate.organisation = owners.organisation
and renovate.repo = owners.repo
where
package_name like 'github.com/oapi-codegen/%'
group by
package_name, version
order by
version asc
This then works as expected:
+------------------------------------+---------+----------+-----------------------------------------------------+
| PACKAGE NAME | VERSION | COUNT(*) | OWNERS |
+------------------------------------+---------+----------+-----------------------------------------------------+
| github.com/deepmap/oapi-codegen | v1.12.4 | 2 | team-one |
| | | | team-two |
| | | | another-team |
| | | | what-about-us |
+------------------------------------+---------+----------+-----------------------------------------------------+